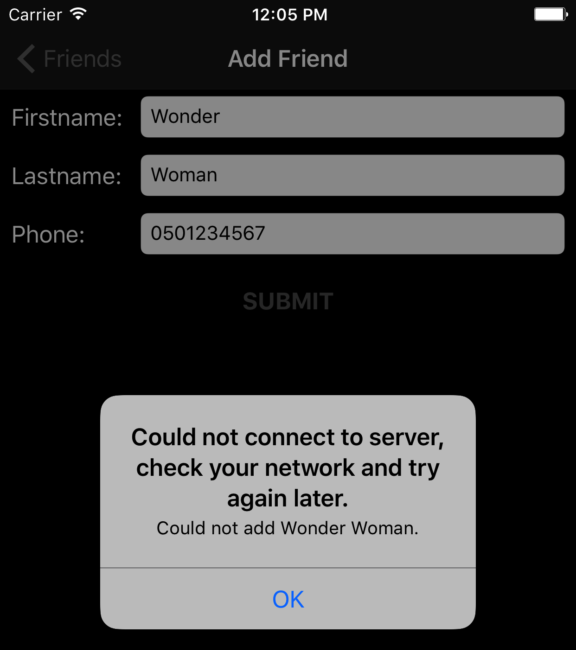
Keep your UI layer clean with viewcontroller extension
This time I wanted talk a little bit about UI layer and how to keep it clean, using UIViewController extensions. UIViewController extensions are an easy way to reduce the lines of code you write inside a viewcontroller. If you can identify a pattern that repeats time and time again in your viewcontrollers, you might want to move that inside an extension. This way, instead of the multiple lines of code for the specific action, you can replace it with a line of code in all your viewcontrollers.
A simple example, about an action that occurs in many of the viewcontrollers, is an error popup note. Often your views are fetching or posting information to a server, and sometimes that interaction fails. Usually in this case, you provide information about the failure to the user using a popup.
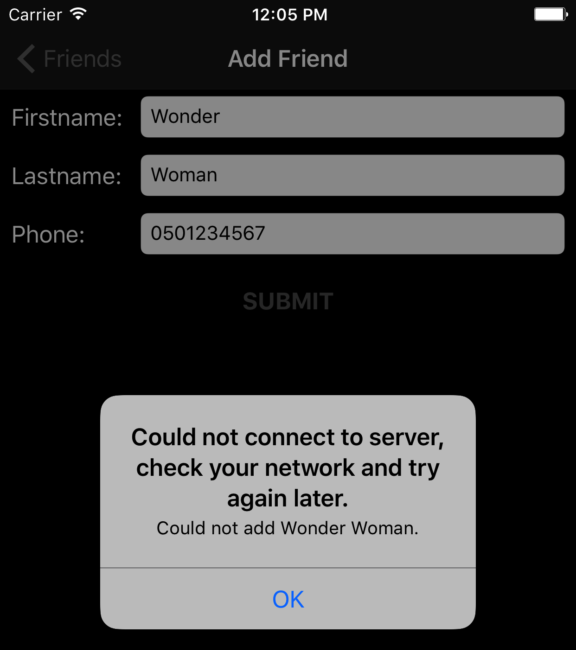
Error popup note
Reduce repeated code with viewcontroller extension
I’ll use the Friend project as a base for this example, so if you want, you can get the complete source code the example from github. Friend application is a mobile app, which allows you to download, add, edit and delete information about your trusted friendlist, which is stored on server. It has a view for listing all the friends from the server, and another view for adding and editing friends information. For more information about the application you can read this blog post.
1 2 3 4 5 6 7 8 9 |
viewModel.onShowError = { [weak self] alert in let alertController = UIAlertController(title: alert.title, message: alert.message, preferredStyle: .alert) alertController.addAction(UIAlertAction(title: alert.action.buttonTitle, style: .default, handler: { _ in alert.action.handler?() })) self.present(alertController, animated: true, completion: nil) } |
Above, you can see the code that creates the AlertController and then uses viewcontrollers present function to make it visible. The fact that both of the viewcontrollers implement this peace of code, violates against the DRY-princible (Don’t Repeat Yourself), so we need to do something about it. Easiest way to fix this, is to define an extension for UIViewController, which then implements these lines.
ViewController extension
Let’s create an UIViewController extension which defines a function called presentSingleButtonAlert(alert: SingleButtonAlert). Inside the function, we’ll put the exact same lines of code that are now used in the viewcontroller:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
import UIKit extension UIViewController { func presentSingleButtonDialog(alert: SingleButtonAlert) { let alertController = UIAlertController(title: alert.title, message: alert.message, preferredStyle: .alert) alertController.addAction(UIAlertAction(title: alert.action.buttonTitle, style: .default, handler: { _ in alert.action.handler?() })) self.present(alertController, animated: true, completion: nil) } } |
Incase you are wondering what the SingleButtonAlert is, it is explained in my previous post in here.
Since presentSingleButtonDialog is defined inside an UIViewController extension, it is now available for all the viewcontrollers through the project. Where ever we used the alert dialog code, we can replace it with the function call. This is what it looks like in the FriendsTableViewController:
1 2 3 |
viewModel?.onShowError = { [weak self] alert in self?.presentSingleButtonDialog(alert: alert) } |
So, we went from 7 lines of code to 1 line of code and incase your project is a lot bigger than Friend project, which it most probably is, you’ll save a lot more lines. At the same time the code becomes easier to maintain. If you need to change the AlertController code, you now only need to do it in one place!
Now this is all very good, but you might not want presentSingleButtonDialog to be automatically available for all your viewcontrollers. Some of them might not need to present dialog. A better way is to define a protocol and make your viewcontroller conform to that protocol.
Protocol extension for viewcontroller
Now, let’s define a new protocol called SingleButtonDialogPresenter, which defines the function presentSingleButtonDialog:
1 2 3 |
protocol SingleButtonDialogPresenter { func presentSingleButtonDialog(alert: SingleButtonAlert) } |
Next, we can create an extension for the protocol and make sure that it is only available for UIViewControllers:
1 2 3 4 5 6 7 8 9 10 11 |
extension SingleButtonDialogPresenter where Self: UIViewController { func presentSingleButtonDialog(alert: SingleButtonAlert) { let alertController = UIAlertController(title: alert.title, message: alert.message, preferredStyle: .alert) alertController.addAction(UIAlertAction(title: alert.action.buttonTitle, style: .default, handler: { _ in alert.action.handler?() })) self.present(alertController, animated: true, completion: nil) } } |
PresentSingleButtonDialog function is identical to the one that was defined inside the extension for UIViewController, but the extension definition is a bit different. It defines that who ever conforms to this protocol, and is also an UIViewController, gets the default implementation for the presentSingleButtonDialog function for free. This is done by setting Self as UIViewController after the where clause.
Now, if we want to use the function in the viewcontroller the viewcontroller needs to conform to SingleButtonPresenter protocol. We can make FriendTableViewController conform to it like this:
1 |
extension FriendsTableViewController: SingleButtonDialogPresenter { } |
Since the default implementation is defined inside the extension, we only have to state in one line that FriendsTableViewController conforms to it, and the function is available.
This was only one simple example, about how to define protocol extension for viewcontroller, but I am sure you will find a lot more use cases where to apply the same technique. Thank you for reading and have a great day my friend!